Oct 11, 2016 Spring Boot Mutual Authentication (2 Way SSL/TLS) Aman Sardana Information Security, Microservices October 11, 2016 February 4, 2017 2 Minutes In one of my earlier articles on cryptographic basics, I discussed about the 3 basic services provided by cryptographic techniques i.e. Confidentiality, integrity and authentication. Sep 10, 2018 This tutorial will demonstrate the process to create user authentication. User login and registration functionality using Spring Security in Spring boot application. User will be able to login. This means that the communication flows from client to API Gateway, and then a separate communication, a separate HTTP request/response, flows from the Apigee Edge API Gateway to the backend (or 'upstream') system. You said that the upstream in your case is a microservices layer, which you implement in Java with Spring Boot. Jan 15, 2019 In this article of REST with Spring,We will see how to build a basic authentication with Spring Security for REST API using Spring Boot. REST API‘s are becoming back bones of many modern enterprise applications.There are multiple choice for the RESTful Authentication.In this article we will build a basic authentication with Spring Security for REST API. I would like to secure the Spring Boot API so it is accessible only for the clients that has valid API key and secret. However, there is no authentication (standard login with username and password) inside the program as all data is anonymous. All I'm trying to achieve is that all API requests can be used only for specific third party front-end.
-->Overview
This article demonstrates creating a Java app with the Spring Initializr that uses the Spring Boot Starter for Azure Active Directory (Azure AD).
- Jan 12, 2020 In a Spring based application, Spring Security is a great authentication and authorization solution, and it provides several options for securing your REST APIs. The simplest approach is utilizing HTTP Basic which is activated by default when you are bootstrap a Spring Boot based application.
- Nov 28, 2016 RESTful API often use GET (read), POST (create), PUT (replace/update) and DELETE (to delete a record). Not all of these are valid choices for every single resource collection, user, or action. Make sure the incoming HTTP method is valid for the session token/API key and associated resource collection, action, and record.
In this tutorial, you learn how to:
- Create a Java application using the Spring Initializr
- Configure Azure Active Directory
- Secure the application with Spring Boot classes and annotations
- Build and test your Java application
If you don't have an Azure subscription, create a free account before you begin.
Prerequisites
The following prerequisites are required in order to complete the steps in this article:
- A supported Java Development Kit (JDK). For more information about the JDKs available for use when developing on Azure, see https://aka.ms/azure-jdks.
- Apache Maven, version 3.0 or later.
Create an app using Spring Initializr
Browse to https://start.spring.io/.
Specify that you want to generate a Maven project with Java, enter the Group and Artifact names for your application.
Scroll down and add Dependencies for Spring Web, Azure Active Directory, and Spring Security.
At the bottom of the page and click the Generate button.
When prompted, download the project to a path on your local computer.
Create Azure Active Directory instance
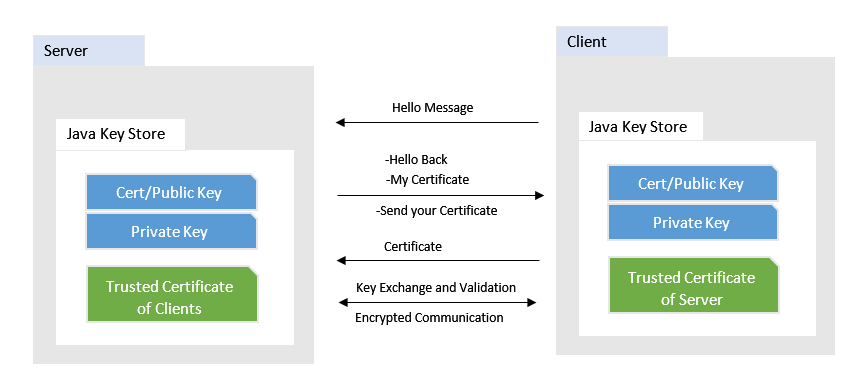
Create the Active Directory instance
Generate An Api Key For Authentication In Spring Boot Windows 10
Log into https://portal.azure.com.
Click +Create a resource, then Identity, and then Azure Active Directory.
Enter your Organization name and your Initial domain name. Copy the full URL of your directory; you will use that to add user accounts later in this tutorial. (For example:
wingtiptoysdirectory.onmicrosoft.com
.)
Copy the full URL of your directory; you will use that to add user accounts later in this tutorial. (For example: wingtiptoysdirectory.onmicrosoft.com.).
When you have finished, click Create. It will take a few minutes to create the new resource.
Generate An Api Key For Authentication In Spring Boot 2017
When complete, click to access the new directory.
Copy the Tenant ID; you will use that value to configure your application.properties file later in this tutorial.
Add an application registration for your Spring Boot app
From the portal menu, click App registrations, and then click Register an application.
Specify your application, and then click Register.
When the page for your app registration appears, copy your Application ID and the Tenant ID; you will use these values to configure your application.properties file later in this tutorial.
Click Certificates & secrets in the left navigation pane. Then click New client secret.
Add a Description and select duration in the Expires list. Click Add. The value for the key will be automatically filled in.
Copy and save the value of the client secret to configure your application.properties file later in this tutorial. (You will not be able to retrieve this value later.)
Click API permissions in the left navigation pane.
On the API permissions page, click Grant admin consent..., and click Yes when prompted.
From the main page for your app registration, click Authentication, and click Add a platform. Then click Web applications.
Enter <http://localhost:8080/login/oauth2/code/azure> as a new Redirect URI, and then click Configure.
From the main page for your app registration, click Manifest, then set the value of the
oauth2AllowImplicitFlow
parameter totrue
, and then click Save.Note
For more information about the
oauth2AllowImplicitFlow
parameter and other application settings, see Azure Active Directory application manifest.
Add a user account to your directory, and add that account to a group
Generate An Api Key For Authentication In Spring Boot For Women
From the Overview page of your Active Directory, click All Users, and then click New user.
When the User panel is displayed, enter the User name and Name. Then click Create.
Note
You need to specify your directory URL from earlier in this tutorial when you enter the user name; for example:
wingtipuser@wingtiptoysdirectory.onmicrosoft.com
Click Groups, then Create a new group that you will use for authorization in your application.
Then click No members selected. (For the purposes of this tutorial, we'll create a group named users.) Search for the user created in the previous step. Click Select to add the user to the group. Then Click Create to create the new group.
Go back to the Users panel, select your test user, and click Reset password, and copy the password; you will use this when you log into your application later in this tutorial.
Configure and compile your app
Extract the files from the project archive you created and downloaded earlier in this tutorial into a directory.
Navigate to the parent folder for your project, and open the
pom.xml
Maven project file in a text editor.Add the dependencies for Spring OAuth2 security to the
pom.xml
:Save and close the pom.xml file.
Navigate to the src/main/resources folder in your project and open the application.properties file in a text editor.
Specify the settings for your app registration using the values you created earlier; for example:
Where:
Parameter Description azure.activedirectory.tenant-id
Contains your Active Directory's Directory ID from earlier. spring.security.oauth2.client.registration.azure.client-id
Contains the Application ID from your app registration that you completed earlier. spring.security.oauth2.client.registration.azure.client-secret
Contains the Value from your app registration key that you completed earlier. azure.activedirectory.active-directory-groups
Contains a list of Active Directory groups to use for authorization. Note
For a full list of values that are available in your application.properties file, see the Azure Active Directory Spring Boot Sample on GitHub.
Save and close the application.properties file.
Create a folder named controller in the Java source folder for your application; for example: src/main/java/com/wingtiptoys/security/controller.
Create a new Java file named HelloController.java in the controller folder and open it in a text editor.
Enter the following code, then save and close the file:
Note
The group name that you specify for the
@PreAuthorize('hasRole(')')
method must contain one of the groups that you specified in theazure.activedirectory.active-directory-groups
field of your application.properties file.You can also specify different authorization settings for different request mappings; for example:
Create a folder named security in the Java source folder for your application; for example: src/main/java/com/wingtiptoys/security/security.
Create a new Java file named WebSecurityConfig.java in the security folder and open it in a text editor.
Enter the following code, then save and close the file:
Build and test your app
Open a command prompt and change directory to the folder where your app's pom.xml file is located.
Build your Spring Boot application with Maven and run it; for example:
After your application is built and started by Maven, open http://localhost:8080 in a web browser; you should be prompted for a user name and password.
Note
You may be prompted to change your password if this is the first login for a new user account.
After you have logged in successfully, you should see the sample 'Hello World' text from the controller.
Note
User accounts which are not authorized will receive an HTTP 403 Unauthorized message.
Summary
Generate An Api Key For Authentication In Spring Boot 2017
In this tutorial, you created a new Java web application using the Azure Active Directory starter, configured a new Azure AD tenant and registered a new application in it, and then configured your application to use the Spring annotations and classes to protect the web app.
Generate An Api Key For Authentication In Spring Boot Program
See also
- For information about new UI options see New Azure portal app registration training guide
Next steps
Generate An Api Key For Authentication In Spring Boots
To learn more about Spring and Azure, continue to the Spring on Azure documentation center.