
importjava.security.Key; |
importjava.security.SecureRandom; |
importjavax.crypto.Cipher; |
importjavax.crypto.KeyGenerator; |
importjavax.crypto.SecretKey; |
importjavax.crypto.spec.IvParameterSpec; |
importorg.apache.commons.codec.binary.Base64; |
publicclassCryptoHelper { |
publicstaticvoidmain( String [] args ) throwsException { |
CryptoHelper crypto =newCryptoHelper(); |
String plaintext ='This is a good secret.'; |
System.out.println( plaintext ); |
String ciphertext = crypto.encrypt( plaintext ); |
System.out.println( ciphertext ); |
String decrypted = crypto.decrypt( ciphertext ); |
System.out.println( decrypted ); |
} |
publicStringencrypt( Stringplaintext ) throwsException { |
return encrypt( generateIV(), plaintext ); |
} |
publicStringencrypt( byte [] iv, Stringplaintext ) throwsException { |
byte [] decrypted = plaintext.getBytes(); |
byte [] encrypted = encrypt( iv, decrypted ); |
StringBuilder ciphertext =newStringBuilder(); |
ciphertext.append( Base64.encodeBase64String( iv ) ); |
ciphertext.append( ':' ); |
ciphertext.append( Base64.encodeBase64String( encrypted ) ); |
return ciphertext.toString(); |
} |
publicStringdecrypt( Stringciphertext ) throwsException { |
String [] parts = ciphertext.split( ':' ); |
byte [] iv =Base64.decodeBase64( parts[0] ); |
byte [] encrypted =Base64.decodeBase64( parts[1] ); |
byte [] decrypted = decrypt( iv, encrypted ); |
returnnewString( decrypted ); |
} |
privateKey key; |
publicCryptoHelper( Keykey ) { |
this.key = key; |
} |
publicCryptoHelper() throwsException { |
this( generateSymmetricKey() ); |
} |
publicKeygetKey() { |
return key; |
} |
publicvoidsetKey( Keykey ) { |
this.key = key; |
} |
publicstaticbyte [] generateIV() { |
SecureRandom random =newSecureRandom(); |
byte [] iv =newbyte [16]; |
random.nextBytes( iv ); |
return iv; |
} |
publicstaticKeygenerateSymmetricKey() throwsException { |
KeyGenerator generator =KeyGenerator.getInstance( 'AES' ); |
SecretKey key = generator.generateKey(); |
return key; |
} |
publicbyte [] encrypt( byte [] iv, byte [] plaintext ) throwsException { |
Cipher cipher =Cipher.getInstance( key.getAlgorithm() +'/CBC/PKCS5Padding' ); |
cipher.init( Cipher.ENCRYPT_MODE, key, newIvParameterSpec( iv ) ); |
return cipher.doFinal( plaintext ); |
} |
publicbyte [] decrypt( byte [] iv, byte [] ciphertext ) throwsException { |
Cipher cipher =Cipher.getInstance( key.getAlgorithm() +'/CBC/PKCS5Padding' ); |
cipher.init( Cipher.DECRYPT_MODE, key, newIvParameterSpec( iv ) ); |
return cipher.doFinal( ciphertext ); |
} |
} |
Two sets of interfaces are included in this package. When a more abstract interface isn't necessary, there are functions for encrypting/decrypting with v1.5/OAEP and signing/verifying with v1.5/PSS. If one needs to abstract over the public-key primitive, the PrivateKey struct implements the Decrypter and Signer interfaces from the crypto package. DecryptPKCS1v15SessionKey is designed for this situation and copies the decrypted, symmetric key (if well-formed) in constant-time over a buffer that contains a random key. Thus, if the RSA result isn't well-formed, the implementation uses a random key in constant time. More specifically, we refer to these types of ciphers as symmetric-key algorithms - meaning that the key, used to encrypt is the same key used to decrypt. Asymmetric Cryptographic Ciphers employ different keys to encrypt and decrypt. In golang, the crypto/rsa package implements such an encryption scheme. C# (CSharp) System.Security.Cryptography AesCryptoServiceProvider.GenerateKey - 30 examples found. These are the top rated real world C# (CSharp) examples of System.Security.Cryptography.AesCryptoServiceProvider.GenerateKey extracted from open source projects. You can rate examples to help us improve the quality of examples. Feb 06, 2017 Symmetric Asymmetric Symmetric Cryptography. Only one key can encrypt. Same key can decrypt. Both the parties need to hold key. Asymmetric Cryptography. Consists of two keys, PUBLIC and PRIVATE; Data encrypted by Private can only be decrypted by Public. Data encrypted by Public can only be decrypted by Private. This was a small description of crypto. Contribute to phylake/go-crypto development by creating an account on GitHub. Golang cryptographic utilities. Contribute to phylake/go-crypto development by creating an account on GitHub. EncryptOAEP (randomSymmetricKey) // decrypt your encrypted symmetric key with your private key randomSymmetricKey2, := privateKey.
commented Dec 25, 2017
Thank you! Thanks to you, I could do this. |
The Go language supports symmetric encryption algorithms in its crypto package. Do not use anything except AES in GCM mode if you don't know what you're doing! Crypto/aes package: AES (Advanced Encryption Standard), also known as Rijndael encryption method, is used by the U.S. Federal government as a block encryption standard.
commented Mar 8, 2018
Brilliant tutorial, just what I have been looking for |
commented Jun 20, 2018
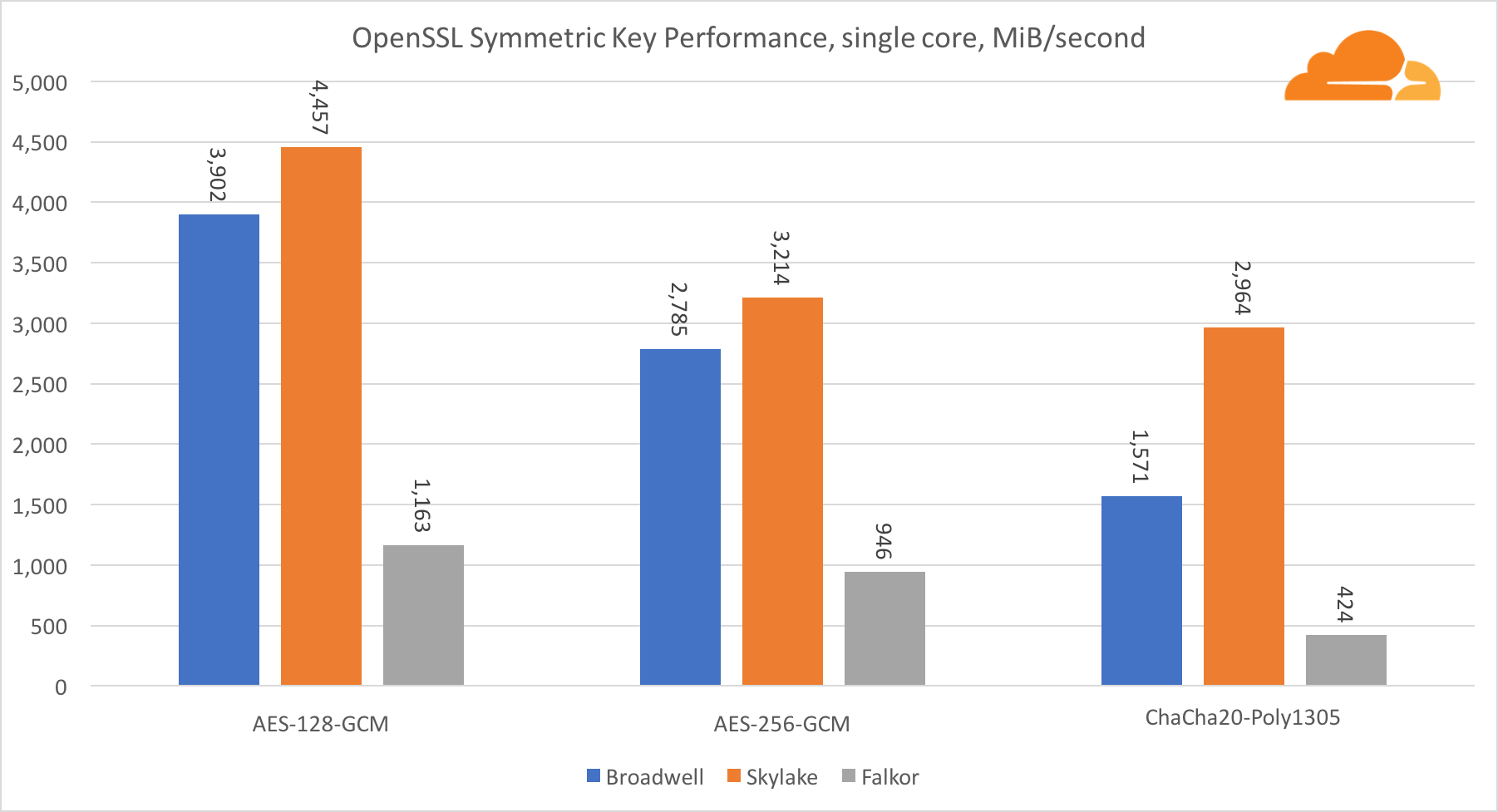
no brilliant tutorial !! |
commented Sep 8, 2018
is this program an example of AES NI implementation? as there are no initialization vectors in NI ..according to my understanding! |
commented Feb 9, 2019
how do you generate the encryption key |
Golang Crypto Tls
// This shows an example of how to generate a SSH RSA Private/Public key pair and save it locally |
package main |
import ( |
'crypto/rand' |
'crypto/rsa' |
'crypto/x509' |
'encoding/pem' |
'golang.org/x/crypto/ssh' |
'io/ioutil' |
'log' |
) |
func main() { |
savePrivateFileTo := './id_rsa_test' |
savePublicFileTo := './id_rsa_test.pub' |
bitSize := 4096 |
privateKey, err := generatePrivateKey(bitSize) |
if err != nil { |
log.Fatal(err.Error()) |
} |
publicKeyBytes, err := generatePublicKey(&privateKey.PublicKey) |
if err != nil { |
log.Fatal(err.Error()) |
} |
privateKeyBytes := encodePrivateKeyToPEM(privateKey) |
err = writeKeyToFile(privateKeyBytes, savePrivateFileTo) |
if err != nil { |
log.Fatal(err.Error()) |
} |
err = writeKeyToFile([]byte(publicKeyBytes), savePublicFileTo) |
if err != nil { |
log.Fatal(err.Error()) |
} |
} |
// generatePrivateKey creates a RSA Private Key of specified byte size |
func generatePrivateKey(bitSize int) (*rsa.PrivateKey, error) { |
// Private Key generation |
privateKey, err := rsa.GenerateKey(rand.Reader, bitSize) |
if err != nil { |
return nil, err |
} |
// Validate Private Key |
err = privateKey.Validate() |
if err != nil { |
return nil, err |
} |
log.Println('Private Key generated') |
return privateKey, nil |
} |
// encodePrivateKeyToPEM encodes Private Key from RSA to PEM format |
func encodePrivateKeyToPEM(privateKey *rsa.PrivateKey) []byte { |
// Get ASN.1 DER format |
privDER := x509.MarshalPKCS1PrivateKey(privateKey) |
// pem.Block |
privBlock := pem.Block{ |
Type: 'RSA PRIVATE KEY', |
Headers: nil, |
Bytes: privDER, |
} |
// Private key in PEM format |
privatePEM := pem.EncodeToMemory(&privBlock) |
return privatePEM |
} |
// generatePublicKey take a rsa.PublicKey and return bytes suitable for writing to .pub file |
// returns in the format 'ssh-rsa ...' |
func generatePublicKey(privatekey *rsa.PublicKey) ([]byte, error) { |
publicRsaKey, err := ssh.NewPublicKey(privatekey) |
if err != nil { |
return nil, err |
} |
pubKeyBytes := ssh.MarshalAuthorizedKey(publicRsaKey) |
log.Println('Public key generated') |
return pubKeyBytes, nil |
} |
// writePemToFile writes keys to a file |
func writeKeyToFile(keyBytes []byte, saveFileTo string) error { |
err := ioutil.WriteFile(saveFileTo, keyBytes, 0600) |
if err != nil { |
return err |
} |
log.Printf('Key saved to: %s', saveFileTo) |
return nil |
} |
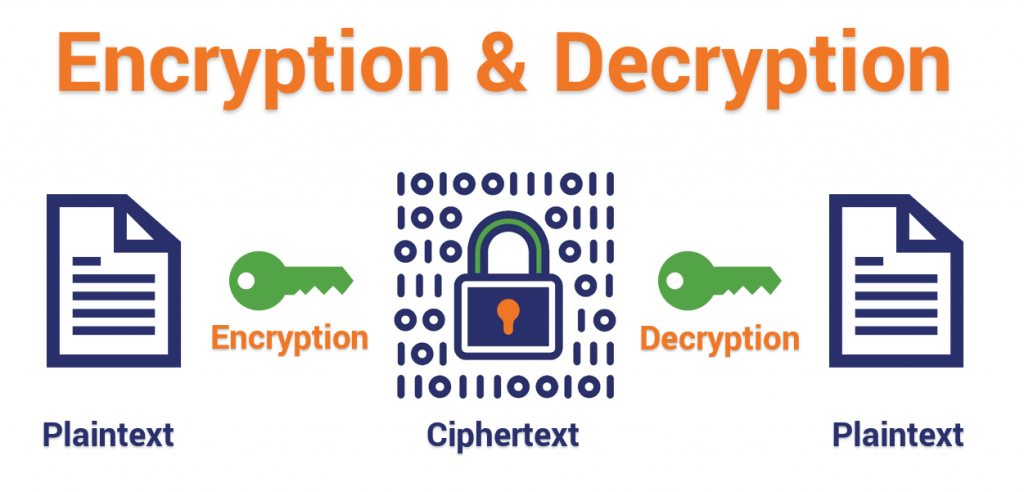